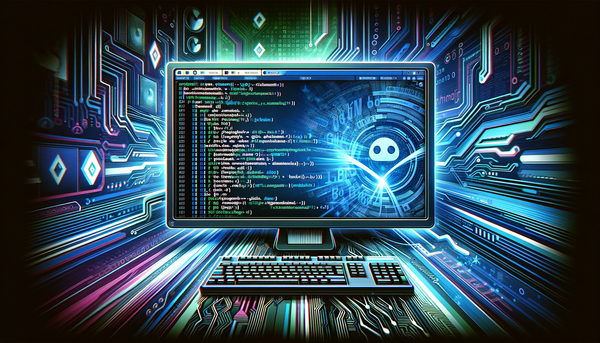
The Ultimate Git Command List: 50 Must-Know Commands for Developers
Here are 50 Git commands that are commonly used in various stages of version control workflow:
Basic Commands
git init
- Initialize a new Git repositorygit clone [url]
- Clone a repository into a new directorygit add [file]
- Add a file to the staging areagit commit -m "[message]"
- Commit changes to the repository with a messagegit status
- Show the working tree statusgit branch
- List, create, or delete branchesgit checkout [branch]
- Switch to a specified branchgit merge [branch]
- Merge a branch into the active branchgit pull [remote] [branch]
- Pull changes from a remote repositorygit push [remote] [branch]
- Push changes to a remote repository
Advanced Branching & Merging
git branch -d [branch]
- Delete a branchgit checkout -b [branch]
- Create and switch to a new branchgit merge --no-ff [branch]
- Merge with a commit even if fast-forward is possiblegit stash
- Stash changes in a dirty working directorygit stash pop
- Apply stashed changes back to the working directory
Inspection and Comparison
git log
- Show commit logsgit log --follow [file]
- Show the commits that changed filegit diff
- Show changes between commits, commit and working tree, etc.git diff --staged
- Show changes between the staging area and the latest commitgit show [commit]
- Show various types of objects
Undoing Changes
git reset [file]
- Unstage a file while retaining the changes in working directorygit reset --hard [commit]
- Reset the staging area and working directory to a specific commitgit revert [commit]
- Revert some existing commitsgit rm [file]
- Remove files from the working tree and the index
Working with Remotes
git remote
- Manage set of tracked repositoriesgit remote add [remote] [url]
- Add a new remotegit fetch [remote]
- Download objects and refs from another repositorygit push [remote] [branch]
- Update remote refs along with associated objectsgit pull
- Fetch from and integrate with another repository or a local branch
Temporary Commits
git stash
- Stash the changes in a dirty working directorygit stash list
- List stack-order of stashed file changesgit stash drop
- Remove a single stashed state from the stash listgit stash clear
- Remove all the stashed states
Tagging
git tag
- List, create, delete or verify a tag object signed with GPGgit tag -a [tag] -m [message]
- Create an annotated taggit push [remote] [tag]
- Share a tag
Git Configurations
git config --global user.name "[name]"
- Define the author name to be used for all commits in the current repo.git config --global user.email "[email address]"
- Define the author email to be used for all commits in the current repo.
Patching
git apply [patch]
- Apply a patch to files and/or to the indexgit cherry-pick [commit]
- Apply the changes introduced by some existing commits
Searching
git grep [string]
- Search for a string in the tracked filesgit bisect
- Use binary search to find the commit that introduced a bug
Submodules
git submodule add [url] [path]
- Add a new submodulegit submodule init
- Initialize a submodulegit submodule update
- Update the submodules
Advanced Git
git rebase [branch]
- Reapply commits on top of another base tipgit rebase --interactive
- Perform an interactive rebasegit filter-branch
- Rewrite branches
Other
git archive
- Create an archive of files from a named treegit gc
- Cleanup unnecessary files and optimize the local repository
These commands cover a wide range of Git functionalities, from basic setup and daily usage to more advanced features like branching, merging, and handling remote repositories.